Canvas How to Upload Photo Larger Than Thumbnail
Paradigm resizing is computationally expensive and usually done on the server-side then that right-sized image files are delivered to the client-side. This arroyo also saves data while transmitting images from the server to the customer.
Nevertheless, there are a couple of situations where you might need to resize images purely using JavaScript on the customer side. For example -
- Resizing images earlier uploading to server
Uploading a large file on your server volition take a lot of time. Y'all can commencement resize images on the browser and then upload them to reduce upload fourth dimension and meliorate application performance.
- Rich image editors that piece of work on client-side
A rich image editor that offers image resize, ingather, rotation, zoom IN and zoom OUT capabilities ofttimes require paradigm manipulation on the client-side. The speed is critical for the user in these editors.
If a user is manipulating a heavy paradigm, it will take a lot of time to download transformed images from the server. Imagine this with operations like undo/redo and complex text and epitome overlays.
Image manipulation in JavaScript is washed using the canvass element. There are libraries like fabric.js that offer rich APIs.
Apart from the above two reasons, in virtually all cases, you would want to get the resized images from the backend itself and then that client doesn't have to bargain with heavy processing tasks.
In this post-
- We will first talk about how to practise resizing purely in JavaScript using the
canvas
element.
- So nosotros volition embrace in bully item how you can resize, crop, and exercise a lot with images past changing the image URL in the
src
attribute. This is the preferred manner to resize images without degrading the user experience programmatically.Also, we will learn how y'all can do this without needing to set upward any libraries or backend servers.
Epitome resizing in JavaScript - Using canvas element
The HTML <canvass>
element is used to draw graphics, on the fly, via JavaScript. Resizing images in browser using sail
is relatively unproblematic.
drawImage
office allows us to render and scale images on canvas element.
drawImage(image, 10, y, width, height)
The offset argument image
tin be created using the Epitome()
constructor, besides equally using any existing <img>
element.
Let's write the code to resize a user-uploaded image on the browser side 300x300
.
<html> <torso> <div> <input blazon="file" id="image-input" have="image/*"> <img id="preview"></img> </div> <script> permit imgInput = certificate.getElementById('prototype-input'); imgInput.addEventListener('change', office (e) { if (e.target.files) { let imageFile = eastward.target.files[0]; var reader = new FileReader(); reader.onload = role (due east) { var img = document.createElement("img"); img.onload = function (event) { // Dynamically create a sail chemical element var sheet = document.createElement("canvas"); // var canvas = certificate.getElementById("canvass"); var ctx = canvas.getContext("2d"); // Actual resizing ctx.drawImage(img, 0, 0, 300, 300); // Show resized paradigm in preview element var dataurl = canvas.toDataURL(imageFile.blazon); certificate.getElementById("preview").src = dataurl; } img.src = eastward.target.upshot; } reader.readAsDataURL(imageFile); } }); </script> </torso> </html>
Let's empathise this in parts. First, the input file type field in HTML
<html> <torso> <div> <input type="file" id="image-input" take = "image/*"> <img id="preview"></img> </div> </body> </html>
Now we need to read the uploaded epitome and create an img
element using Epitome()
constructor.
let imgInput = document.getElementById('image-input'); imgInput.addEventListener('modify', function (east) { if (e.target.files) { let imageFile = e.target.files[0]; var reader = new FileReader(); reader.onload = role (e) { var img = document.createElement("img"); img.onload = function(issue) { // Actual resizing } img.src = e.target.result; } reader.readAsDataURL(imageFile); } });
Finally, let's draw the prototype on canvas and show preview element.
// Dynamically create a canvas element var canvas = document.createElement("canvas"); var ctx = canvas.getContext("2d"); // Bodily resizing ctx.drawImage(img, 0, 0, 300, 300); // Show resized image in preview element var dataurl = canvas.toDataURL(imageFile.type); document.getElementById("preview").src = dataurl;
You might notice that the resized image looks distorted in a few cases. It is considering we are forced 300x300
dimensions. Instead, we should ideally only manipulate i dimension, i.e., height or width, and adapt the other accordingly.
All this can be done in JavaScript, since you accept access to input image original pinnacle (img.width
) and width using (img.width
).
For example, we tin can fit the output paradigm in a container of 300x300
dimension.
var MAX_WIDTH = 300; var MAX_HEIGHT = 300; var width = img.width; var height = img.height; // Change the resizing logic if (width > height) { if (width > MAX_WIDTH) { height = peak * (MAX_WIDTH / width); width = MAX_WIDTH; } } else { if (elevation > MAX_HEIGHT) { width = width * (MAX_HEIGHT / height); height = MAX_HEIGHT; } } var canvas = certificate.createElement("canvas"); canvas.width = width; canvas.height = height; var ctx = canvas.getContext("2d"); ctx.drawImage(img, 0, 0, width, height);
Decision-making epitome scaling behavior
Scaling images can result in fuzzy or blocky artifacts. There is a trade-off betwixt speed and quality. By default browsers are tuned for meliorate speed and provides minimum configuration options.
You lot tin can play with the following properties to control smoothing effect:
ctx.mozImageSmoothingEnabled = faux; ctx.webkitImageSmoothingEnabled = simulated; ctx.msImageSmoothingEnabled = false; ctx.imageSmoothingEnabled = simulated;
Image resizing in JavaScript - The serverless way
ImageKit allows you to dispense image dimensions direct from the epitome URL and get the exact size or crop y'all want in existent-time. Kickoff with a single master image, as large as possible, and create multiple variants from the aforementioned.
For example, we tin create a 400 x 300
variant from the original image similar this:
https://ik.imagekit.io/ikmedia/ik_ecom/shoe.jpeg?tr=w-400,h-300
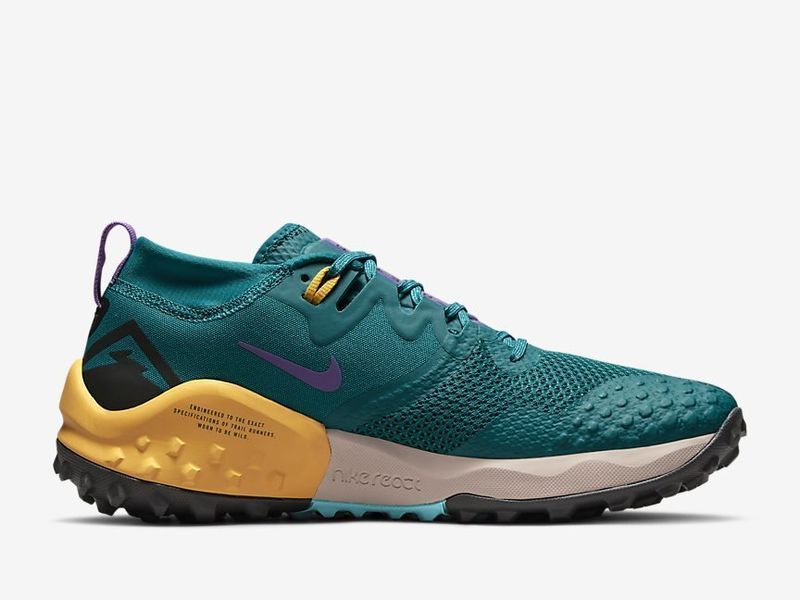
Yous can apply this URL directly on your website or app for the product epitome, and your users get the correct image instantly.
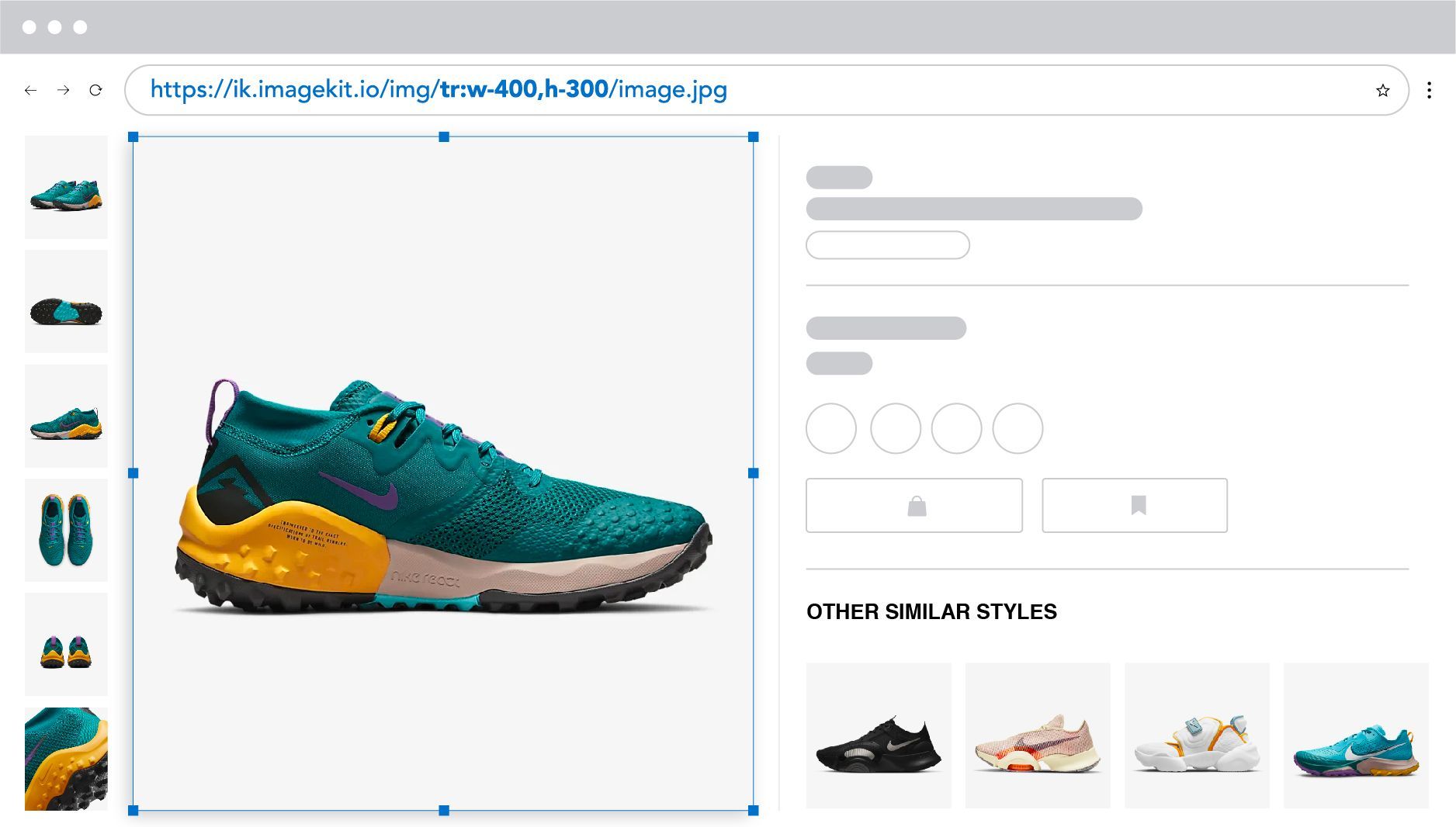
If you don't want to crop the prototype while resizing, at that place are several possible crop modes.
https://ik.imagekit.io/ikmedia/ik_ecom/shoe.jpeg?tr=w-400,h-300,cm-pad_resize,bg-F5F5F5
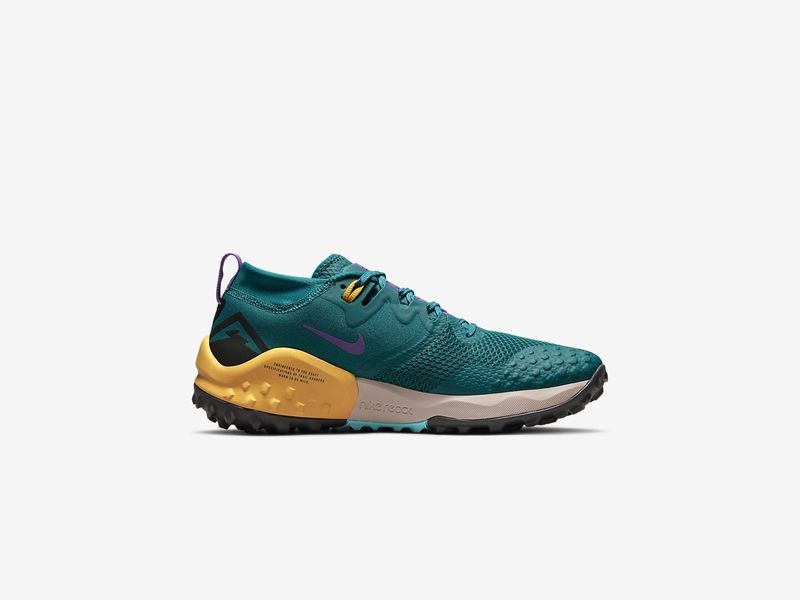
We have published guides on how you tin exercise the post-obit things using ImageKit'due south real-time image manipulation.
- Resize image - Basic height & width manipulation
- Cropping & preserving the aspect ratio
- Face up and object detection
- Add together a watermark
- Add a text overlay
- Arrange for tedious internet connectedness
- Loading a blurred low-quality placeholder
Summary
- In most cases, you should not do paradigm resizing in the browser because information technology is deadening and results in poor quality. Instead, you should use an epitome CDN like ImageKit.io to resize images dynamically by changing the paradigm URL. Try our forever complimentary plan today!
- If your apply-case demands client-side resizing, information technology is possible using the
canvas
element.
Source: https://imagekit.io/blog/how-to-resize-image-in-javascript/
0 Response to "Canvas How to Upload Photo Larger Than Thumbnail"
Post a Comment